Rails ActionMailbox with Postal
We love using open source and self hosted applications and Postal is one of these great tools we use at Lcx. But how can you use the new Rails ActionMailbox with Postal?
What is Postal?
Postal is an "A fully featured open-source mail delivery platform for incoming & outgoing e-mail" or as it says on the GitHub page
Postal is a complete and fully featured mail server for use by websites & web servers. Think Sendgrid, Mailgun or Postmark but open-source and ready for you to run on your own servers. Postal was developed by aTech Media to serve its own mail processing requirements and we have since decided that it should be released as an open-source project for the community.
I like using Postal since it runs on our own servers and it simplifies GDPR to not have yet another data processor, it also simplifies creating lots of different mail accounts for all kind of Apps and other tools we use.
Setting up ActionMailbox for Postal
I'm not going into the setup of ActionMailbox, if that's what you are looking for you can look at this Video from GoRails
Setup the Controller
You first need a new controller to process mails coming from Postal, create a new file app/controllers/action_mailbox/ingresses/postal/inbound_emails_controller.rb
# frozen_string_literal: true
module ActionMailbox
class Ingresses::Postal::InboundEmailsController < ActionMailbox::BaseController
#before_action :authenticate_by_password
def create
ActionMailbox::InboundEmail.create_and_extract_message_id! Base64.decode64(params.require("message"))
rescue ActionController::ParameterMissing => error
logger.error <<~MESSAGE
#{error.message}
MESSAGE
head :unprocessable_entity
end
end
end
As you can see I commented out the before_action :authenticate_by_password
which is potentially a bad idea, but I did not get to test why postal is not sending the password. There are potential solutions to this like checking the IP from which the request comes but more on that in a future post.
The Important part is the Base64.decode64(params.require("message"))
since Postal will send the message Base64 encoded and that won't really work.
Now fire up ngrok to create a possibility to receive emails from external and add this to your development.rb
config.hosts << "*****.ngrok.io"
config.action_mailbox.ingress = :postal
Set the correct host instead of ***.ngrok.io
otherwise Rails 6 will refuse the request.
Now with your App running and reachable from the outside via ngrok, it's time that you configure postal.
Configuring Postal
Create an Endpoint
Go to your postal server -> routing -> HTTP Endpoints
Set the URL to your ngrok URL https://***.ngrok.io/rails/action_mailbox/postal/inbound_emails
and configure the other options as you like it, the encoding doesn't really matter, both options worked fine with Action Mailbox.
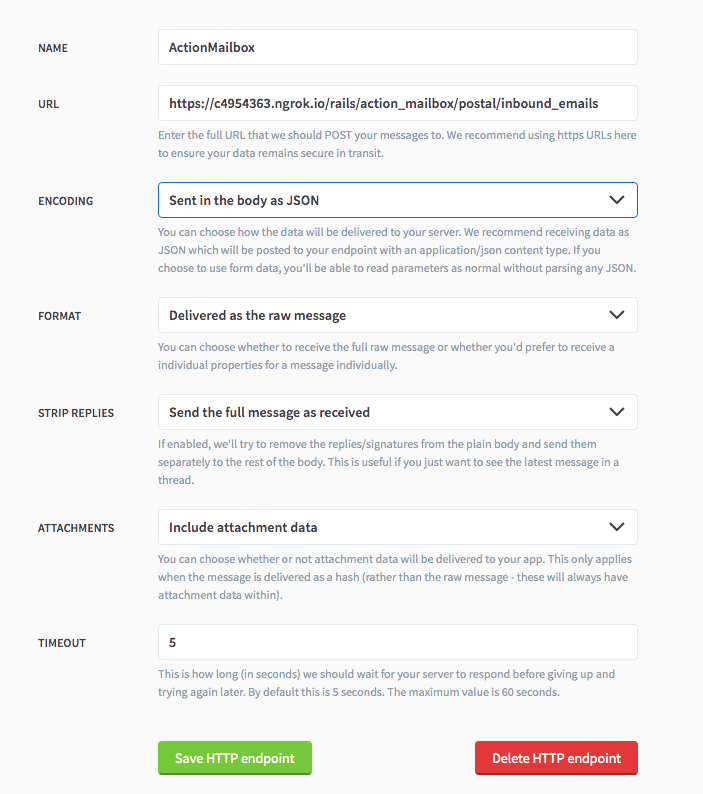
Create a new route
Now create a new route in Postal using the HTTP Endpoint you just created
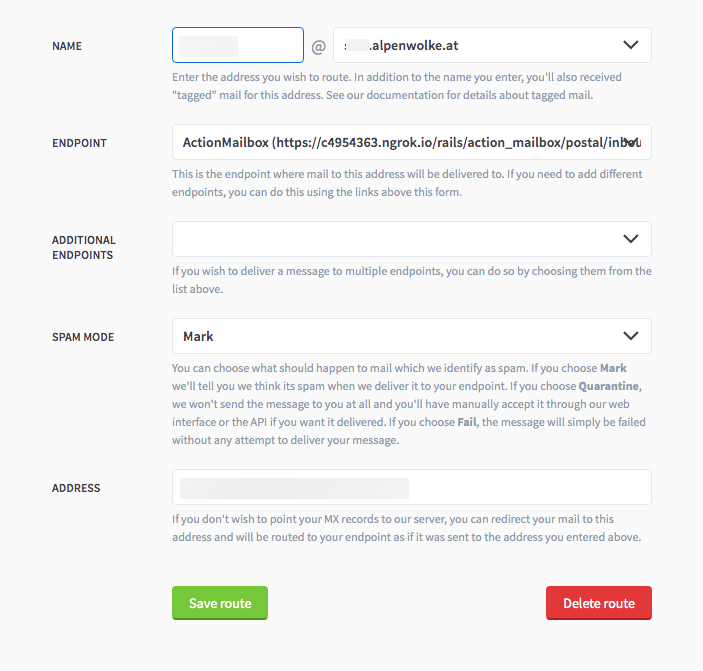
What will happen now is that if you send a mail to this newly created mailbox, assuming the MX Records point to your postal server, it will be routed via ngrok to your Rails App with ActionMailbox!
All of this self-hosted, GDPR safe and open source!
Let me know if this article helped you setup postal with ActionMailbox