Thingsboard with Raspberry and Sensehat
It's hot outside, we added air conditioning to our office and now of course we all want to know if it helps in any way. Of course we can feel it in the office but we can't measure it. So let's fix that.
Thingsboard
We could have gone with a thermometer from the hardware store, it would be cheap and fast but no fun at all. So let's look at the more fun version of what we are building.
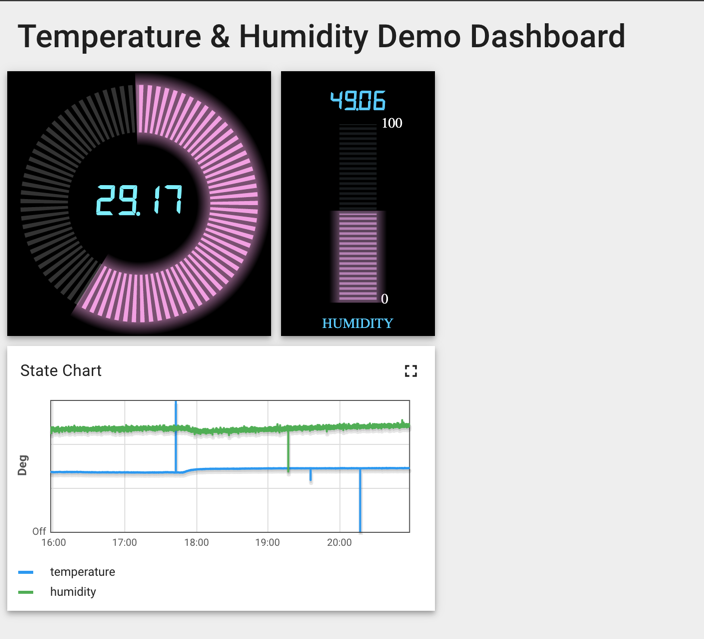
Setup
First, you need a running thingsboard server, the easiest way to do that is by using docker.
docker run -it -p 9090:9090 -p 1883:1883 -p 5683:5683/udp -v /opt/docker/thingsboard/data:/data -v /opt/docker/thingsboard/logs:/var/log/thingsboard --name mytb --restart always thingsboard/tb-postgres
If you are hooking up a reverse proxy in front of thingsboard you need to add some additional configuration for the websockets to work
location /api/ws {
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection "Upgrade";
proxy_set_header CLIENT_IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
proxy_read_timeout 86400;
proxy_pass http://thingsboard/api/ws;
}
After setting this up, add a new device as tenant admin in thingsboard.
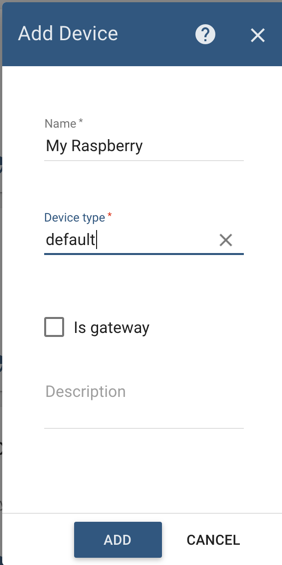
After adding the device go to dashboard and edit the existing "Temperature & Humidity Demo Dashboard" and remove the existing DHT11 device and add the raspberry you just created, do this for both temperature and humidity sensors.
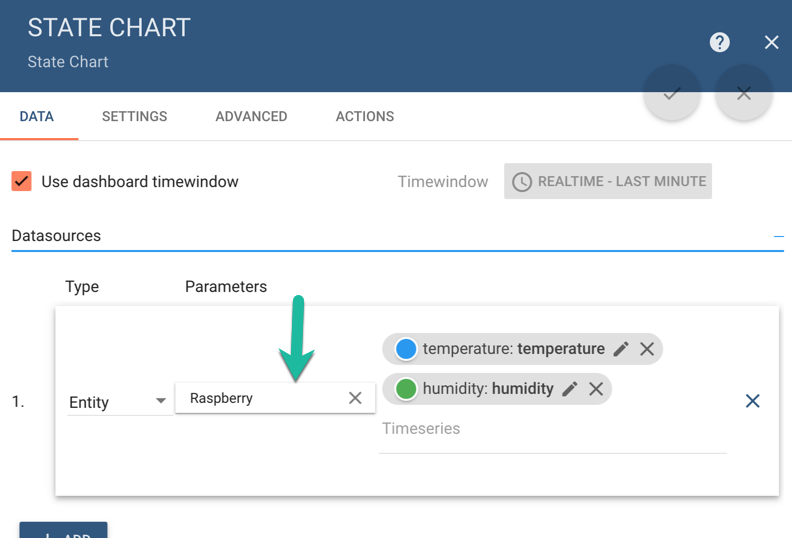
Check out the thingsboard documentation for more details.
Raspberry setup
I had a sense hat laying around the office so I used that to gather temperature and humidity.
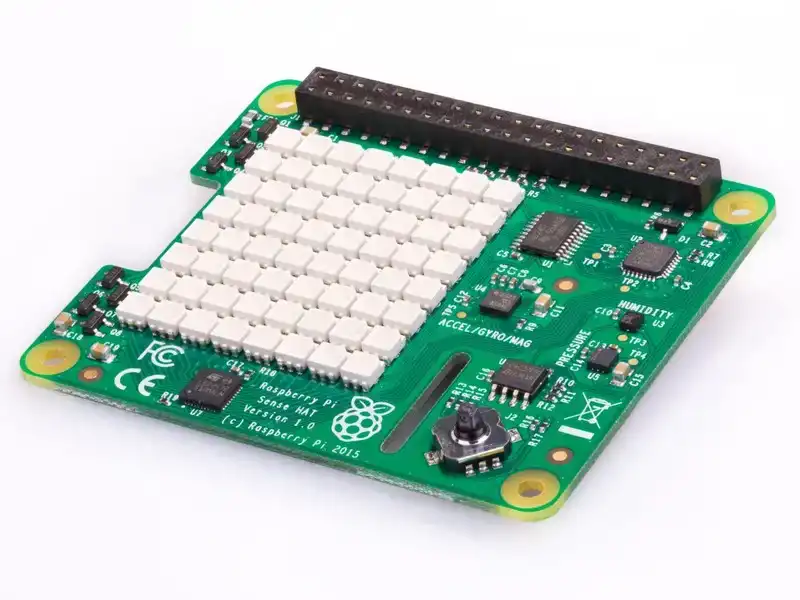
Install the required sense hat package.
sudo apt-get install sense-hat
Then create this python script and replace **IP**
with the IP of your thingsboard installation and also replace ACCESSTOKENw
with the access token from your device.
import os
import time
import sys
import paho.mqtt.client as mqtt
import json
from sense_hat import SenseHat
THINGSBOARD_HOST = '**IP**'
ACCESS_TOKEN = '**ACCESSTOKEN**'
INTERVAL=2
sense = SenseHat()
sensor_data = {'temperature': 0, 'humidity': 0}
next_reading = time.time()
client = mqtt.Client()
# Set access token
client.username_pw_set(ACCESS_TOKEN)
# Connect to ThingsBoard using default MQTT port and 60 seconds keepalive interval
client.connect(THINGSBOARD_HOST, 1883, 60)
client.loop_start()
try:
while True:
humidity = sense.get_humidity()
temperature = sense.get_temperature()
humidity = round(humidity, 2)
temperature = round(temperature, 2)
print(u"Temperature: {:g}, Humidity: {:g}%".format(temperature, humidity))
sensor_data['temperature'] = temperature
sensor_data['humidity'] = humidity
# Sending humidity and temperature data to ThingsBoard
client.publish('v1/devices/me/telemetry', json.dumps(sensor_data), 1)
next_reading += INTERVAL
sleep_time = next_reading-time.time()
if sleep_time > 0:
time.sleep(sleep_time)
except KeyboardInterrupt:
pass
client.loop_stop()
client.disconnect()
Run the script and you are done! Your raspberry will be sending data to thingsboard.
Now you probably wish you went with the cheap and quick thermometer from the hardware store.