Using the sunsky api with ruby
Sunsky offers an API, unfortunately only php and java example code exists and communication with sunsky can be tricky (with the people, not the API).
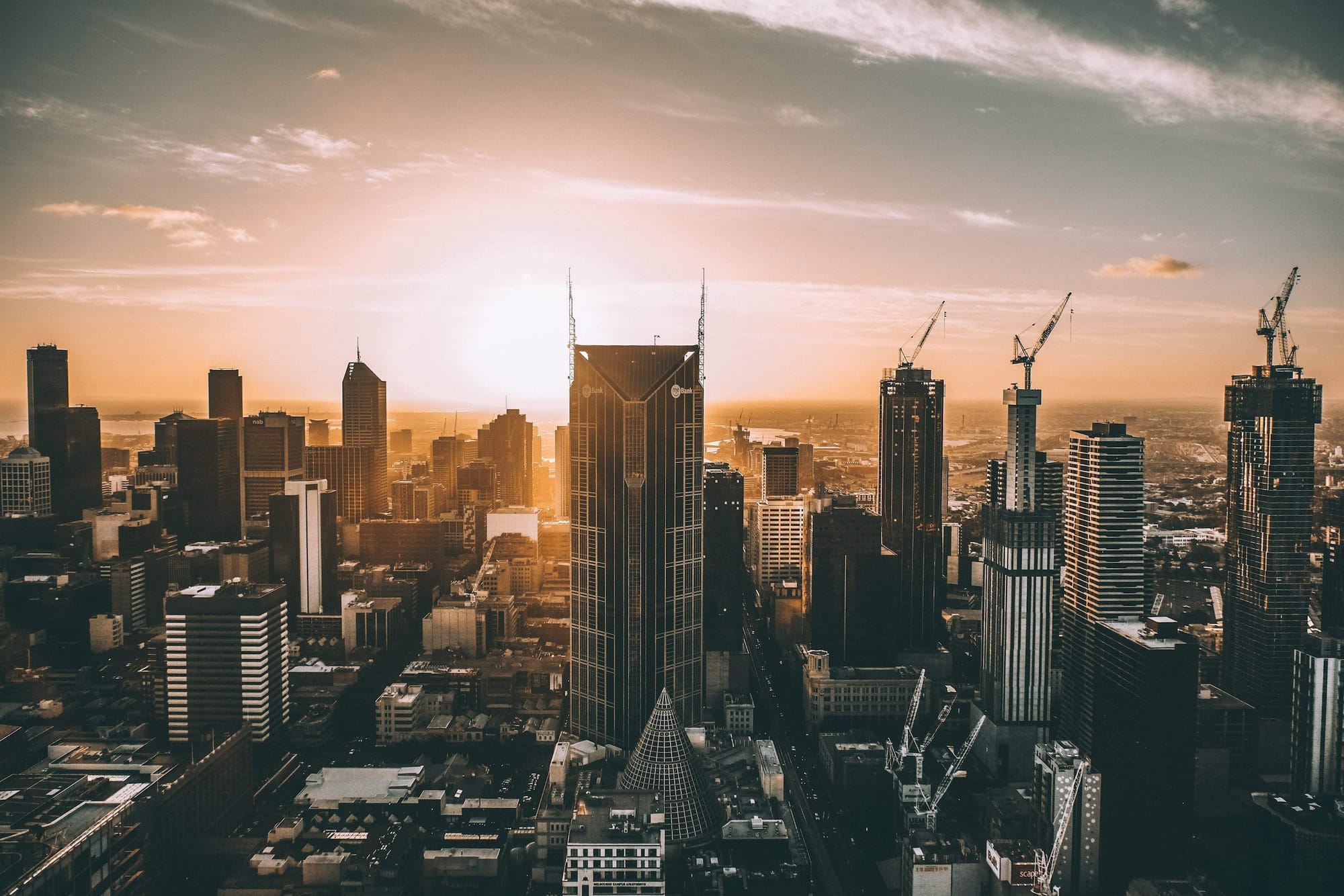
The api is pretty simple to understand looking at the example PHP code. Each request needs to send the signed parameters. The signature is a simple md5 with the secret at the end. The important thing is that the keys are sorted alphabeticaly!
def sign(parameters,secret)
signature=''
parameters.sort.each {|key,value|signature+=value}
signature+="@#{secret}"
Digest::MD5.hexdigest(signature)
end
then there is the call and download function which are basicaly the same, only difference is that the download function saves a zip file.
def call(api_url,secret,parameters)
signature=sign(parameters,secret)
parameters['signature'] = signature
uri = URI.parse(api_url)
response = Net::HTTP.post_form(uri, parameters)
response.body
end
for the download, we can reuse the call method and just dump the data to a file
def download(api_url, secret, parameters, path)
data=call(api_url,secret,parameters)
File.open(path, 'w') { |file| file.write(data) }
end
putting it all together
require 'digest'
require 'net/http'
def sign(parameters,secret)
signature=''
parameters.sort.each {|key,value|signature+=value}
signature+="@#{secret}"
Digest::MD5.hexdigest(signature)
end
def call(api_url,secret,parameters)
signature=sign(parameters,secret)
parameters['signature'] = signature
uri = URI.parse(api_url)
response = Net::HTTP.post_form(uri, parameters)
response.body
end
def download(api_url, secret, parameters, path)
data=call(api_url,secret,parameters)
File.open(path, 'w') { |file| file.write(data) }
end
key = "MYKEY";
secret = "VERYSECRET";
api_url="http://www.sunsky-api.com/openapi/product!getImages.do"
parameters={
'key' =>key,
'itemNo' => 'S-SCS-2850W'
}
download(api_url, secret, parameters, "foo.zip")